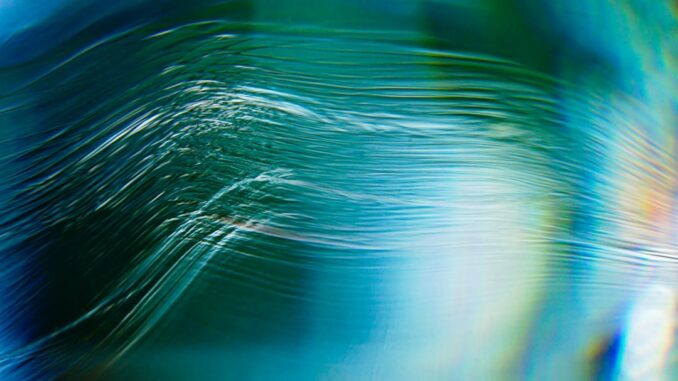
Abstract
Algorithms are the bedrock of modern computation, underpinning everything from simple search queries to complex artificial intelligence systems. This report delves into the multifaceted world of algorithms, providing an in-depth exploration suitable for experts in the field. We begin by revisiting fundamental concepts, examining algorithm design paradigms, and analyzing computational complexity. We then move to the cutting edge, investigating advanced algorithmic techniques for large-scale data processing, machine learning, and optimization. Finally, we address the critical ethical considerations arising from the pervasive deployment of algorithms in society, including bias, fairness, transparency, and accountability. This report aims to provide a comprehensive overview, highlighting both the remarkable power and the potential pitfalls of algorithmic innovation.
Many thanks to our sponsor Esdebe who helped us prepare this research report.
1. Introduction
Algorithms, at their core, are well-defined sequences of instructions designed to solve a specific problem or accomplish a particular task. While the concept of an algorithm predates the advent of electronic computers – Euclid’s algorithm for finding the greatest common divisor being a prime example – the digital age has propelled algorithms into a position of unprecedented importance. They are no longer merely theoretical constructs but rather practical tools that shape our daily lives, driving everything from search engines and social media platforms to financial markets and autonomous vehicles.
The ongoing revolution in artificial intelligence (AI) has further intensified the focus on algorithms. Machine learning (ML) algorithms, in particular, have demonstrated remarkable capabilities in areas such as image recognition, natural language processing, and predictive modeling. However, the increasing complexity and opaqueness of these algorithms have also raised serious concerns about their potential for bias, unfairness, and lack of transparency. Therefore, a thorough understanding of algorithms, their limitations, and their ethical implications is essential for researchers, practitioners, and policymakers alike.
This report aims to provide a comprehensive exploration of algorithms, encompassing their theoretical foundations, practical applications, and ethical considerations. We will examine key algorithmic design paradigms, analyze their computational complexity, and delve into advanced techniques for handling large-scale data and complex problems. Furthermore, we will critically evaluate the ethical challenges posed by the widespread deployment of algorithms in society, offering insights and recommendations for responsible algorithmic innovation.
Many thanks to our sponsor Esdebe who helped us prepare this research report.
2. Foundations of Algorithm Design and Analysis
2.1 Algorithmic Design Paradigms
Algorithm design is a creative process, but it is guided by established paradigms that offer systematic approaches to problem-solving. These paradigms provide a framework for developing efficient and effective algorithms, enabling us to tackle a wide range of computational challenges. Some of the most prominent algorithmic design paradigms include:
-
Divide and Conquer: This paradigm involves breaking down a problem into smaller subproblems, solving each subproblem recursively, and then combining the solutions to obtain the solution to the original problem. Examples include Merge Sort and Quick Sort, which achieve efficient sorting by recursively dividing the input array into smaller subarrays.
-
Dynamic Programming: This approach is used for optimization problems where the optimal solution can be constructed from optimal solutions to overlapping subproblems. Dynamic programming avoids recomputing solutions to subproblems by storing them in a table or memoization structure. Classic examples include the Fibonacci sequence and the shortest path problem (e.g., using the Bellman-Ford algorithm).
-
Greedy Algorithms: These algorithms make locally optimal choices at each step with the hope of finding a globally optimal solution. Greedy algorithms are often simpler and more efficient than dynamic programming, but they do not always guarantee an optimal solution. Examples include Dijkstra’s algorithm for finding the shortest path in a graph and Huffman coding for data compression.
-
Backtracking: This paradigm is used for solving constraint satisfaction problems by systematically searching for solutions. Backtracking explores possible solutions incrementally, abandoning partial solutions that violate the constraints. The N-Queens problem and Sudoku solvers are typical applications of backtracking.
-
Branch and Bound: An improvement over backtracking, this technique is often applied to optimization problems. By calculating bounds on possible solutions, it can prune branches of the search tree that are guaranteed not to lead to an optimal solution, often dramatically reducing the search space.
Selecting the appropriate algorithmic design paradigm is crucial for developing efficient and effective algorithms. The choice depends on the specific problem characteristics, the desired level of optimality, and the available computational resources.
2.2 Computational Complexity Analysis
Computational complexity analysis provides a framework for evaluating the efficiency of algorithms. It focuses on quantifying the resources required by an algorithm, such as time and space, as a function of the input size. This analysis allows us to compare different algorithms for the same problem and choose the most efficient one.
The most common measure of computational complexity is Big O notation, which describes the asymptotic upper bound on the growth rate of the algorithm’s resource usage. For example, an algorithm with a time complexity of O(n) has a running time that grows linearly with the input size n, while an algorithm with a time complexity of O(n^2) has a running time that grows quadratically with n.
Understanding computational complexity is essential for designing algorithms that can handle large-scale data and complex problems. Algorithms with exponential time complexity, such as O(2^n) or O(n!), are generally impractical for large inputs, while algorithms with polynomial time complexity, such as O(n), O(n log n), or O(n^2), are considered more efficient.
Beyond Big O notation, other measures of complexity exist. Big Omega (Ω) describes the asymptotic lower bound, and Big Theta (Θ) describes the tight asymptotic bound (both upper and lower). Furthermore, amortized analysis provides an average-case complexity over a sequence of operations, which can be useful for understanding the performance of data structures that occasionally perform expensive operations.
2.3 NP-Completeness and Approximation Algorithms
Some problems are inherently difficult to solve efficiently. NP-complete problems are a class of problems for which no known polynomial-time algorithm exists. If a polynomial-time algorithm were found for any NP-complete problem, it would imply that all problems in the NP class can be solved in polynomial time, which is a major unsolved problem in computer science (the P versus NP problem).
Examples of NP-complete problems include the Traveling Salesperson Problem (TSP), the Knapsack Problem, and the Boolean Satisfiability Problem (SAT). Since finding exact solutions to NP-complete problems is often computationally infeasible for large inputs, researchers have developed approximation algorithms that aim to find near-optimal solutions in a reasonable amount of time.
Approximation algorithms provide a trade-off between solution quality and computational efficiency. They guarantee that the solution found is within a certain factor of the optimal solution. The approximation ratio is a measure of the quality of the approximation algorithm. For example, a 2-approximation algorithm for the TSP guarantees that the solution found is no more than twice the length of the optimal solution.
Many thanks to our sponsor Esdebe who helped us prepare this research report.
3. Advanced Algorithmic Techniques
3.1 Algorithms for Large-Scale Data Processing
The era of big data has presented new challenges and opportunities for algorithm design. Traditional algorithms that work well on small datasets may become inefficient or even infeasible when applied to large-scale data. Therefore, researchers have developed specialized algorithmic techniques for processing massive datasets efficiently. Some of these techniques include:
-
MapReduce: This programming model and framework allows for parallel processing of large datasets across a distributed cluster of computers. MapReduce divides the input data into smaller chunks, processes each chunk independently in parallel, and then combines the results to obtain the final output. Hadoop is a popular open-source implementation of MapReduce.
-
Streaming Algorithms: These algorithms process data sequentially, one element at a time, without storing the entire dataset in memory. Streaming algorithms are particularly useful for processing data streams, such as network traffic or sensor data. Examples include Bloom filters for membership testing and reservoir sampling for selecting a random sample from a stream.
-
Locality-Sensitive Hashing (LSH): This technique is used for finding approximate nearest neighbors in high-dimensional spaces. LSH hashes similar data points to the same bucket with high probability, allowing for efficient similarity search. LSH is widely used in information retrieval, recommendation systems, and data mining.
-
Sketching Algorithms: These algorithms create compact summaries of large datasets, allowing for efficient approximate queries. Examples include Count-Min sketches for estimating the frequency of items in a stream and HyperLogLog for estimating the cardinality of a set.
3.2 Algorithms for Machine Learning
Machine learning algorithms are at the heart of modern AI systems. These algorithms learn from data and make predictions or decisions without being explicitly programmed. Some of the most widely used machine learning algorithms include:
-
Supervised Learning: This type of learning involves training a model on a labeled dataset, where each data point is associated with a known output or target variable. Examples include linear regression, logistic regression, support vector machines (SVMs), and decision trees.
-
Unsupervised Learning: This type of learning involves training a model on an unlabeled dataset, where the goal is to discover hidden patterns or structures in the data. Examples include clustering algorithms (e.g., k-means, hierarchical clustering), dimensionality reduction techniques (e.g., principal component analysis (PCA), t-distributed stochastic neighbor embedding (t-SNE)), and anomaly detection algorithms.
-
Reinforcement Learning: This type of learning involves training an agent to make decisions in an environment in order to maximize a reward signal. Reinforcement learning algorithms are used in robotics, game playing, and control systems. Examples include Q-learning and deep reinforcement learning (e.g., using deep neural networks to approximate the Q-function).
-
Deep Learning: A subfield of machine learning that uses artificial neural networks with multiple layers (deep neural networks) to learn complex representations of data. Deep learning has achieved remarkable success in areas such as image recognition, natural language processing, and speech recognition. Convolutional neural networks (CNNs) are commonly used for image processing, while recurrent neural networks (RNNs) and transformers are used for sequence data processing.
3.3 Optimization Algorithms
Optimization algorithms are used to find the best solution to a problem from a set of possible solutions. These algorithms are widely used in engineering, finance, and computer science. Some of the most common optimization algorithms include:
-
Gradient Descent: This iterative algorithm is used to find the minimum of a function by repeatedly moving in the direction of the negative gradient. Gradient descent is widely used in training machine learning models.
-
Newton’s Method: This algorithm uses the first and second derivatives of a function to find its minimum. Newton’s method converges faster than gradient descent but requires computing the second derivative, which can be computationally expensive.
-
Evolutionary Algorithms: These algorithms are inspired by biological evolution and use concepts such as selection, crossover, and mutation to find the best solution to a problem. Genetic algorithms are a popular example of evolutionary algorithms.
-
Simulated Annealing: This algorithm is inspired by the annealing process in metallurgy and uses a probabilistic approach to find the global optimum of a function. Simulated annealing is particularly useful for optimization problems with many local optima.
Many thanks to our sponsor Esdebe who helped us prepare this research report.
4. Ethical Considerations and Challenges
4.1 Bias and Fairness
Algorithms can perpetuate and amplify existing biases in data, leading to unfair or discriminatory outcomes. This is particularly concerning in applications such as criminal justice, loan applications, and hiring, where algorithmic decisions can have a significant impact on people’s lives.
Bias can arise from various sources, including biased training data, biased algorithm design, and biased evaluation metrics. Addressing bias requires careful attention to data collection, algorithm development, and evaluation. Techniques such as fairness-aware machine learning, data augmentation, and bias detection can help mitigate bias in algorithms.
Furthermore, defining fairness itself is a complex issue. Different notions of fairness exist, such as statistical parity, equal opportunity, and predictive parity. Choosing the appropriate fairness metric depends on the specific application and the values and priorities of the stakeholders involved. Trade-offs between different fairness metrics often exist; improving one metric may worsen another. This necessitates careful consideration of the potential consequences of different algorithmic choices.
4.2 Transparency and Interpretability
The increasing complexity of algorithms, particularly deep learning models, has made it difficult to understand how these algorithms make decisions. This lack of transparency and interpretability can erode trust in algorithmic systems and make it difficult to identify and correct errors or biases.
Explainable AI (XAI) is a growing field that aims to develop techniques for making algorithms more transparent and interpretable. XAI techniques include feature importance analysis, rule extraction, and visualization. These techniques can help users understand which features are most influential in the algorithm’s decisions and how the algorithm arrives at its conclusions.
However, achieving full transparency and interpretability can be challenging, especially for complex algorithms. Furthermore, there is a trade-off between accuracy and interpretability. Simpler, more interpretable algorithms may be less accurate than complex, opaque algorithms. Finding the right balance between accuracy and interpretability is crucial for building trustworthy and responsible algorithmic systems.
4.3 Accountability and Responsibility
The widespread deployment of algorithms raises questions about accountability and responsibility. Who is responsible when an algorithm makes a mistake or causes harm? Is it the algorithm designer, the data provider, the user, or someone else?
Establishing clear lines of accountability and responsibility is essential for ensuring that algorithms are used ethically and responsibly. This requires developing legal and regulatory frameworks that address the specific challenges posed by algorithmic systems. Furthermore, it requires fostering a culture of ethical awareness and responsibility among algorithm designers, developers, and users.
One approach is to implement auditing and monitoring mechanisms to detect and correct errors or biases in algorithmic systems. Another approach is to establish independent oversight bodies that can review and evaluate algorithmic systems before they are deployed. Ultimately, ensuring accountability requires a multi-faceted approach that involves technical, legal, and ethical considerations.
4.4 Privacy and Data Security
Algorithms often rely on large amounts of data, which may contain sensitive personal information. Protecting the privacy and security of this data is crucial for building trustworthy algorithmic systems. Data breaches and privacy violations can erode public trust and have serious consequences for individuals and organizations.
Techniques such as anonymization, differential privacy, and secure multi-party computation can help protect the privacy of data used in algorithmic systems. Anonymization removes identifying information from data, while differential privacy adds noise to the data to prevent individual data points from being identified. Secure multi-party computation allows multiple parties to compute a function on their private data without revealing the data to each other.
In addition to technical measures, legal and regulatory frameworks such as the General Data Protection Regulation (GDPR) are essential for protecting privacy. These frameworks establish rules and guidelines for the collection, processing, and use of personal data.
Many thanks to our sponsor Esdebe who helped us prepare this research report.
5. Future Directions
The field of algorithms is constantly evolving, with new challenges and opportunities emerging on the horizon. Some key areas of future research and development include:
-
Quantum Algorithms: Quantum computers promise to solve certain problems much faster than classical computers. Developing quantum algorithms for problems such as drug discovery, materials science, and cryptography is a major area of research.
-
Federated Learning: This approach allows machine learning models to be trained on decentralized data sources without sharing the data itself. Federated learning is particularly useful for privacy-sensitive applications, such as healthcare and finance.
-
Neuromorphic Computing: This type of computing is inspired by the structure and function of the human brain. Neuromorphic computers offer the potential for energy-efficient and highly parallel computation.
-
Explainable and Responsible AI: Developing more transparent, interpretable, and fair algorithms is a crucial area of research. This includes developing new XAI techniques, fairness-aware machine learning algorithms, and methods for detecting and mitigating bias in algorithms.
-
AI Safety: Ensuring that AI systems are safe and aligned with human values is a critical challenge. This includes developing methods for verifying and validating AI systems, preventing unintended consequences, and ensuring that AI systems are used ethically and responsibly.
Many thanks to our sponsor Esdebe who helped us prepare this research report.
6. Conclusion
Algorithms are fundamental to modern computation and play an increasingly important role in our lives. Understanding the principles of algorithm design and analysis, as well as the ethical considerations associated with their deployment, is essential for researchers, practitioners, and policymakers alike.
This report has provided a comprehensive overview of algorithms, encompassing their theoretical foundations, practical applications, and ethical challenges. We have examined key algorithmic design paradigms, analyzed their computational complexity, and explored advanced techniques for handling large-scale data and complex problems. Furthermore, we have critically evaluated the ethical implications of algorithms, offering insights and recommendations for responsible algorithmic innovation.
As algorithms continue to evolve and shape our world, it is crucial to remain vigilant about their potential impacts and to strive for algorithms that are not only efficient and effective but also fair, transparent, and accountable. By embracing a responsible and ethical approach to algorithmic innovation, we can harness the power of algorithms for the benefit of society as a whole.
Many thanks to our sponsor Esdebe who helped us prepare this research report.
References
- Cormen, T. H., Leiserson, C. E., Rivest, R. L., & Stein, C. (2009). Introduction to Algorithms (3rd ed.). MIT Press.
- Bishop, C. M. (2006). Pattern Recognition and Machine Learning. Springer.
- Goodfellow, I., Bengio, Y., & Courville, A. (2016). Deep Learning. MIT Press.
- Shalev-Shwartz, S., & Ben-David, S. (2014). Understanding Machine Learning: From Theory to Algorithms. Cambridge University Press.
- Aggarwal, C. C. (2018). Data Mining: The Textbook. Springer.
- O’Neil, C. (2016). Weapons of Math Destruction: How Big Data Increases Inequality and Threatens Democracy. Crown.
- Barocas, S., Hardt, M., & Narayanan, A. (2019). Fairness and Machine Learning: Limitations and Opportunities. MIT Press.
- Rudin, C. (2019). Stop explaining black box machine learning models for high stakes decisions and use interpretable models instead. Nature Machine Intelligence, 1(5), 206-215.
- Provost, F., & Fawcett, T. (2013). Data Science for Business: What You Need to Know About Data Mining and Data-Analytic Thinking. O’Reilly Media.
- Domingos, P. (2015). The Master Algorithm: How the Quest for the Ultimate Learning Machine Will Remake Our World. Basic Books.
Be the first to comment